23. Enumeration types#
Picture this: You’re programming a traffic light system. You have three colors to work with: red, yellow, and green. How would you represent these in your code? You could use integers, where 0
is red, 1
is yellow, and 2
is green. Or you could use strings, like "red"
,"yellow"
, "green"
.
Both approaches would work, but they’re not particularly intuitive or safe. Someone reading your code might not immediately understand what the numbers or strings represent.
Said differently, the set of possible values of string
or int
is not the same as that of a traffic light.
The value "apple"
is for example a valid string
but not a valid traffic light state.
This is where enums, short for enumerations or enumeration types, come into play in C#.
An enumeration is a distinct type that consists of a set of named constants. It provides a way to define a type that can have one of a few distinct values, and those values can have names that are meaningful to your code.
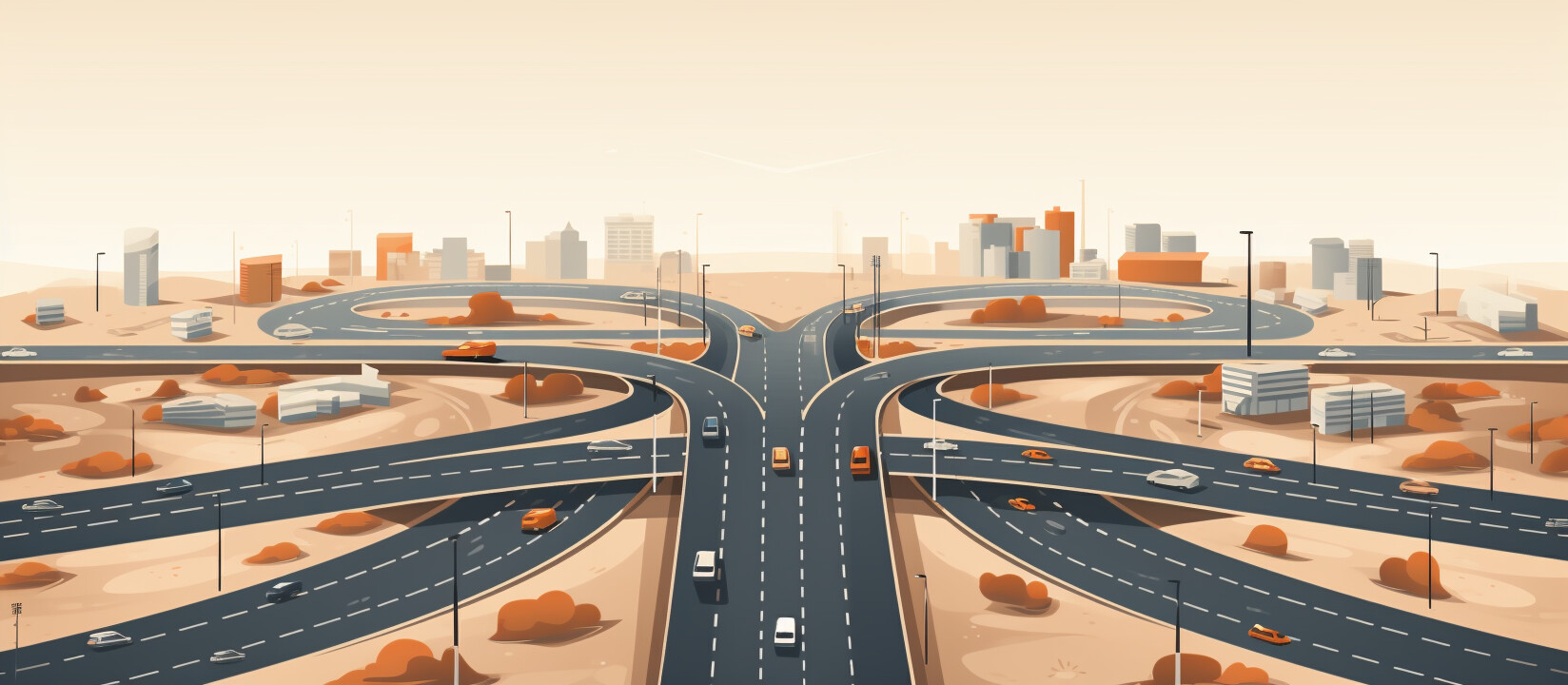
Fig. 23.1 A traffic light whose states we can enumerate (red, yellow, green) is a splendid example of when enumeration types are useful.#
Here’s how you could define an enumeration for our traffic light colors:
enum TrafficLightColor
{
Red,
Yellow,
Green
}
In this example, TrafficLightColor
is an enumeration that has three possible values: Red
, Yellow
, and Green
.
Now, you can define a variable of type TrafficLightColor
, and assign it one of the values:
TrafficLightColor currentColor = TrafficLightColor.Red;
This makes your code more readable because it’s clear what values currentColor
can have. More importantly, it makes your code more safe, because you someone cannot accidentally assign an invalid value to currentColor
.
currentColor = TrafficLightColor.Orange;
(1,34): error CS0117: 'TrafficLightColor' does not contain a definition for 'Orange'
By default, the underlying type of an enumeration is int
, and the constants are assigned values starting from 0
and increasing by 1
for each item. In our example, Red
would have a value of 0
, Yellow
a value of 1
, and Green
a value of 2
. You can explicitly specify the underlying type and values for the constants when defining the enumeration if you want:
enum TrafficLightColor : byte
{
Red = 1,
Yellow = 2,
Green = 3
}
In this case, Red
would have a value of 1
, Yellow
a value of 2
, and Green
a value of 3
, and the underlying type of the enumeration is byte
.
Enumerations enhance the readability, safety, and organization of your code. They’re especially useful when a variable can only take one out of a small set of possible values. There are plenty of cases where enums are useful, but some obvious ones include weekdays and months.
By the way, you’ve now created your first custom data type. You’re on a roll, let’s keep going.