8. Variables#
Imagine that you’ve got a kitchen cabinet full of glass or porcelain jars and lots of different items to store. To keep track of the contents you put labels on the jars. In the world of programming, these jars are what we call variables. The label on the jar is the variable name, and the item you put inside the jar is the value.
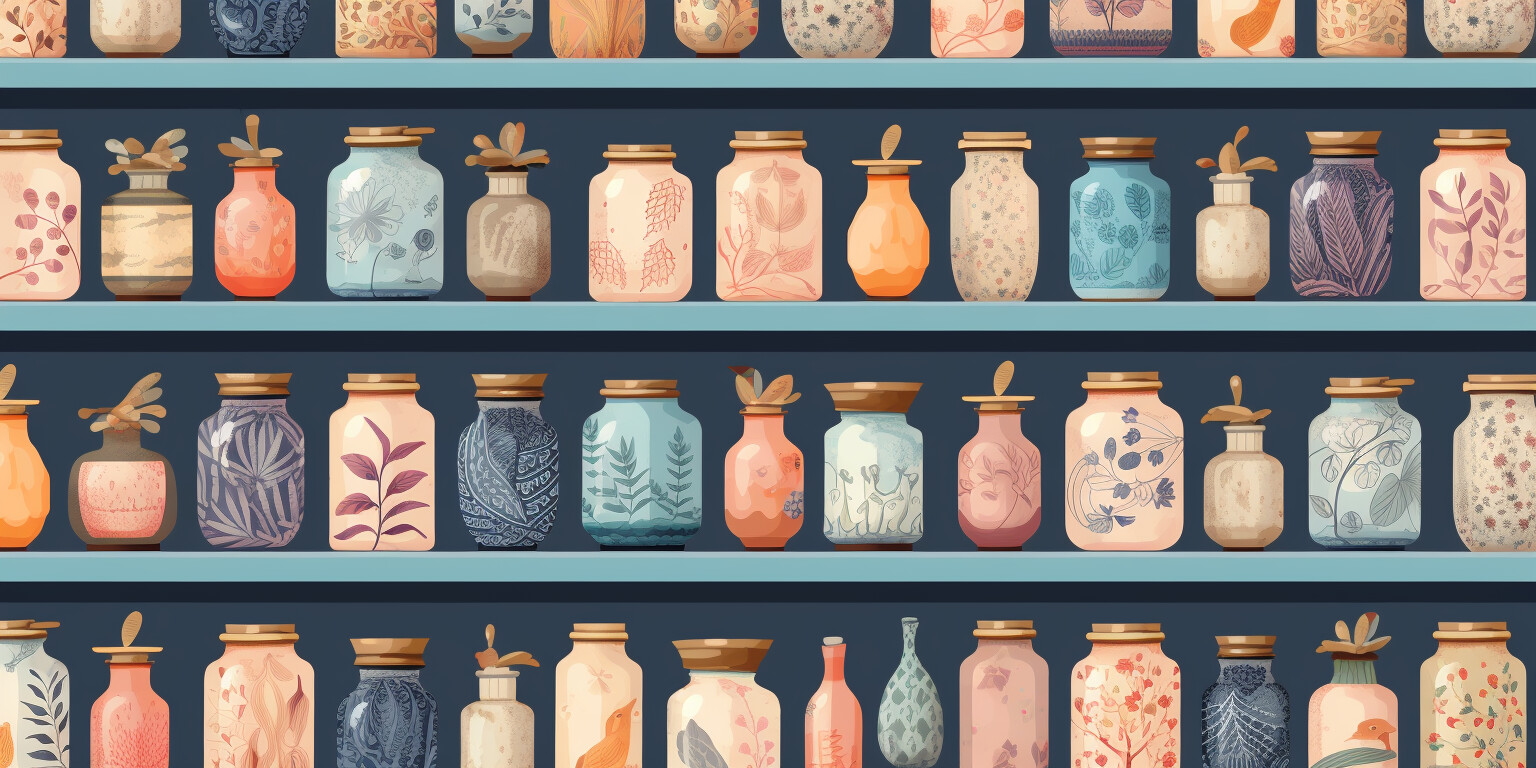
Fig. 8.1 A variable is like a jar, a variable name like a label, and a value its content.#
Declaring a variable is like adding an empty jar with a label to the shelf. In C#, you declare a variable by stating its type (more on types later) and name. For example, the line int myNumber;
declares a variable named myNumber
of type int
. This is like adding a jar and slapping a ‘myNumber’ label on it. The int
tells us what kind of items can be stored in this jar – in this case, integer numbers - meaning whole numbers.
// Declaring a variable with a type and a name.
// Putting a new jar on the shelf and labling it.
int myNumber;
When you have your labeled jar ready, you can put something in it. This is called assignment. For instance, if you write myNumber = 10;
you’re assigning the value 10
to the variable myNumber
. This is like putting an item into your labeled jar. The item here is the number 10
.
// Assigning a value to a variable.
// Putting some contents into the jar.
myNumber = 10;
Often, you’ll want to declare a variable and assign a value to it at the same time. This is known as initialization. If you write int myNumber = 10;
you’re declaring a variable and initializing it with a value in one go. This is like getting a new container, labeling it, and putting an item in it right away.
// Initializing a variable.
// Putting a new jar on the shelf, labling it, and adding some contents all in one go.
int myNumber = 10;
One important thing to note is the difference between an undeclared variable and an uninitialized one. An undeclared variable is like a container without a label – the program doesn’t know it exists. An uninitialized variable, on the other hand, is a labeled container that doesn’t have anything in it yet. It exists, but it’s empty.
If a variable called yourNumber
is undeclared and you try to use it then you will get the following error:
The name 'yourNumber' does not exist in the current context (CS0103)
If the variable is unassigned then you will instead get the following error:
Use of unassigned local variable 'yourNumber'
Now, what makes variables particularly interesting in programming is that in some languages (such as C#) their values can change, or in other words, they are ‘variable’. This is why they’re called variables! Think back to our shelf of jars analogy. Just because you put one item in a jar, it doesn’t mean that it has to stay there forever. You can take out the value, put it in another jar or throw it away, and then put a new item in the jar. The same goes for variables in programming.
Take this line of code, for example: myNumber = 20;
. If we have previously assigned myNumber a value of 10
, this new line doesn’t cause an error. Instead, it simply changes the value stored in myNumber
from 10
to 20
. We say that the variable myNumber
is mutable, meaning it can be changed.
This leads us to an important point about the equal sign (=
) in programming. Unlike in mathematics, where it denotes equality, in programming, it denotes assignment. It’s more like an arrow pointing from the right to the left, indicating the direction of assignment. So, when you see myNumber = 20;
you should read it as “myNumber gets the value of 20”, rather than “myNumber equals 20”. The value on the right is assigned to the variable on the left. This distinction is essential to correctly understanding and using variables in programming.
Tip
We often talk about the “left-hand side” and “right-hand side” so it is wise to become accustomed to this terminology.
You’ve learned about declaring, assigning, and initializing variables. You’ve learned the difference between an undeclared and an uninitialized variable. Finally, you’ve learned about the meaning of the equal sign in programming and about the mutable nature of variables. With these foundations, we’re now ready to you’re ready to delve deeper into C# programming in the following chapters. Happy coding!